7 Must Have Basic Tools for TypeScript Modules
The easiest part of development is the first written code. The difficult parts are the planning phase and maintaining the code. Your planning skills will improve over time. By using the fundamental tools in this list, you can make your code maintenance easier right now.
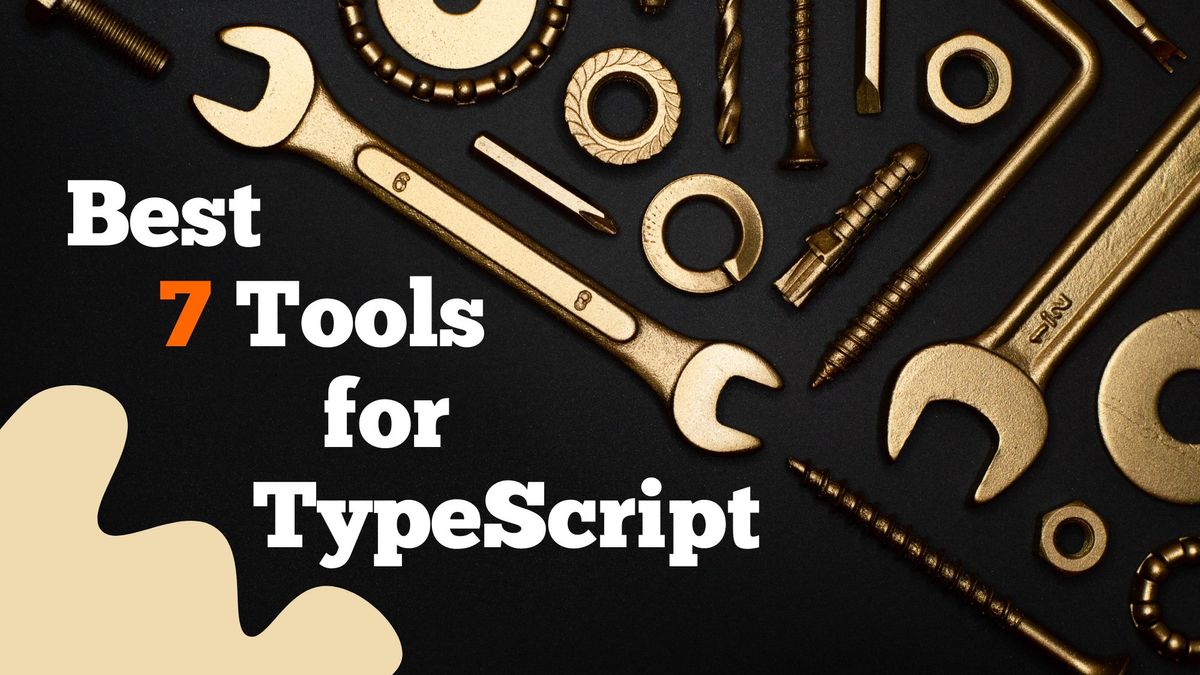
If you would like to setup all the tools with zero-configuration, try this free and open source npm package as below.
$ mkdir my-project
$ npm init @ozum/tsm@latest
Like every ecosystem, Node.js has its unique philosophy and common beliefs. At the forefront of these are small modules developed to do one thing exceptionally well. Like many Node.js developers, if you're also developing numerous small modules and publishing them on npm, the following libraries and tools will greatly simplify your life. In this list, I've included the essential tools. In my next article, I'll list advanced tools that complement this set.
You can use these tools individually, but after properly configuring all the tools in both lists, it's almost impossible to publish faulty code.
Below, I've listed these tools for those who are curious, and I use them as well.
1. ESLint
ESLint statically analyzes your JavaScript or TypeScript code and informs you about problematic areas. While ESLint can catch some formatting errors, the consensus is that it's more appropriate to use the next tool for that purpose.
2. Prettier
There are no two developers who entirely agree on code formatting. Sometimes, you might even debate with your own inner voice about how to format the code. Your opinion might change depending on whether you got up on the right or left side of the bed that day. Prettier can put an end to all these issues. Prettier automatically formats various languages, primarily JavaScript and TypeScript.
3. EditorConfig
Every editor comes with its own set of configurations, and if your team members are using a different editor than you, some settings may not align with the project's general principles. EditorConfig, designed to solve this problem, automatically applies the most basic settings for all widely-used editors.
4. Jest / Vitest
The most crucial stage of software development is not writing code but writing tests. Yes, I know you also attach great importance to tests. While the JavaScript and TypeScript ecosystem hosts numerous testing tools, one of the most popular is Jest. Beginners may find it challenging to configure the current Jest (v29) version with TypeScript and ES Modules. In such cases, it's beneficial to take a look at Vitest, which provides out-of-the-box support for TypeScript and ES Modules.
5. Knip
This tool might not be as popular as the others at the time of writing this article, but the list would be incomplete without mentioning it. Knip is a tool that finds unused files, exports, and dependencies in your project. Like all the other tools in this list, I use this one too and want to thank the developer, Lars, for his caring (read: patience) in responding to all my questions on GitHub, no matter how illogical they may be.
6. TypeDoc
Who writes documentation? Everyone, right? TypeDoc eliminates all your excuses for not writing documentation for your library's API. It generates documentation in HTML or Markdown format (with the Markdown plugin) based on API information written in a subset of JSDoc comments you include in your code. My advice is to write your descriptions in everyday language. For example:
/**
* Adds given two numbers.
*
* @param a is the first number to add.
* @param b is the second number to add.
* @returns sum of the two numbers
*
* @example
* const total = add(1, 4);
*/
function add(a: number, b: number): number {}
7. swpm
We can consider this tool a hidden gem. If you're using package managers like npm
, yarn
, pnpm
, etc., you can't assume that other users are using the same package manager as you. This issue could be crucial enough to prevent some modules from functioning. swpm (Switch Package Manager) allows you to run various package manager commands independently of the package manager being used.
For instance, the swpm install
command will execute either npm install
, yarn add
, or pnpm install
, depending on the user's preference.
You can check out the next post to learn about more advanced tools as well.
Comments ()