Set Default Parameter Value for JavaScript Function
Learn how and why to set default parameter values in JavaScript functions. See examples for named and positioned parameters.
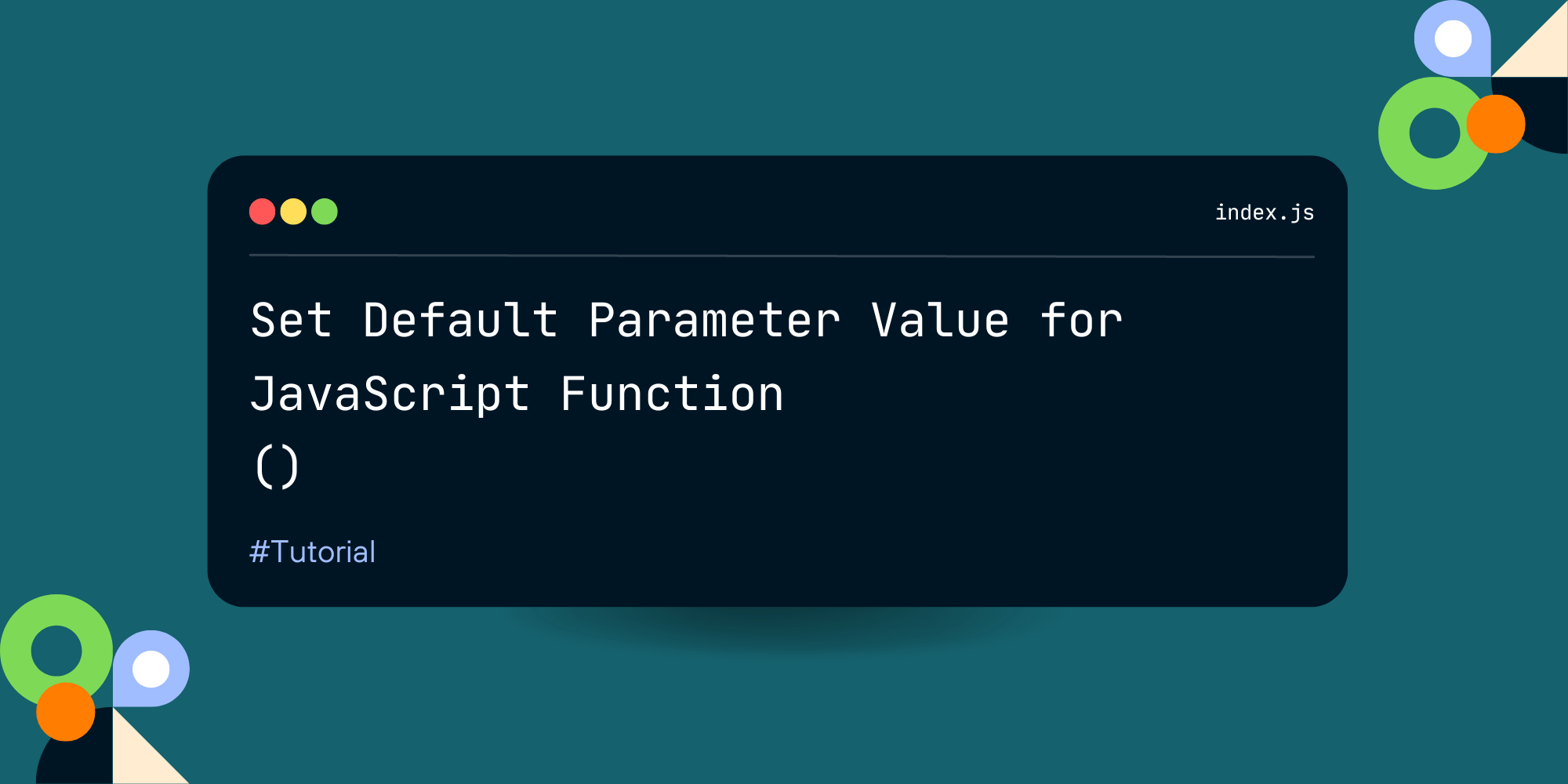
TL;DR
JavaScript default parameters are optional parameters having the default value of the parameter in the function definition. If we don't pass a value or pass an undefined value, the default value expression is used:
// Example #1: JavaScript function with a positioned parameter
// and a default parameter value.
function greet(message = "Hello World!") {
console.info(message);
}
greet(); // Hello World
greet("Hola Mars"); // Hola Mars
// Example #2: JavaScript function with named parameters
// and default parameter values.
function greet({ salutation = "Hello", name = "World" }) {
console.info(`${salutation} ${name}`);
}
greet(); // ERROR: Cannot read properties of undefined. See Example #3
greet({}); // Hello World
greet({ salutation: "Hola", name: "Mars" }); // Hola Mars
// Example #3: JavaScript function with named parameters,
// default parameter values and a default parameter value for the whole positioned object.
function greet({ salutation = "Hello", name = "World" } = {}) {
console.info(`${salutation} ${name}`);
}
greet(); // Hello World
greet({}); // Hello World
greet({ salutation: "Hola", name: "Mars" }); // // Hola Mars
Parameters and Arguments: What is the difference?
I (and some other developers, maybe you) use parameter and argument terms interchangeably, and my friends and colleagues understand what I mean. Technically, parameters are the names of the function arguments we defined in the function declaration. Arguments are the actual values we passed to the functions. See the below example:
function greet(greeting, name) {
// Function body
}
greet("Hello", "World");
In the above example, greeting
and name
are the parameters of the greet()
function. Hello
and World
are the argument values.
How to Set Default Function Parameters
In JavaScript, all parameters have an undefined
value as a default parameter value. See the following example:
function greet(salutation, name) {}
The above code is equal to the below code:
function greet(salutation = undefined, name = undefined) {}
If I want to provide a different default value other than the undefined
, I will explicitly define it like the following example:
function greet(salutation = "Hello", name) {
const space = name === undefined ? "" : " ";
const who = name ?? "";
console.info(`${salutation}${space}${who}`);
}
I provide Hello
default value for the first parameter and make it an optional parameter but leave the second parameter without a default value. In the body of the function, I checked whether the name is defined to avoid Hello undefined
message. I use the ternary operator (?
) as a conditional statement to decide whether I need a space based on the second parameter's value and a nullish coalescing operator (??
) to assign an empty string as the default value of the parameter for the greeted thing. Please note, ??
only checks for an undefined
value and a null
value but not falsy values. You can see a better solution for the above code. I'd better provide a default second value for the function declaration, as in the following example:
function greet(salutation = "Hello", name = "") {
const space = name === undefined ? "" : " ";
console.info(`${salutation}${space}${name}`);
}
We don't have to use primitive values for the default function parameters. In the next section, I will show you how to use an expression.
Default Value Expression
We can use complex expressions for the parameter's default value to decide the default behavior of the function. One of the available options is using another function in the function signatures, as in the below example:
let LANGUAGE;
const GREETING = {
en: "Hello",
tr: "Merhaba",
es: "Hola",
};
function setLanguage(language) {
LANGUAGE = language;
}
function getSalutation() {
return GREETING[LANGUAGE ?? "en"];
}
function greet(salutation = getSalutation(), name = "World") {
console.info(`${salutation} ${name}`);
}
setLanguage("es");
greet(); // Hola World
setLanguage("en");
greet(); // Hello World
The JavaScript engine evaluates argument values at the call time. In the above example,
In the first function call, I set the language Spanish and then use the default parameter value based on the language I set. At the call time, language equals es
, and the result is Hola World
. In the second function call, the language is English, and the result is Hello World
.
Using a Destructured Parameter in Optional Parameters
In my opinion, object destructuring is one of the most excellent features added to the programming language. It is a simple syntactic sugar to assign multiple variables easily.
const { name, color } = { name: "Pencil", color: "Red", id: "xyz" };
console.info( `${name} is ${color}`); // Pencil is Red
The restructured values depend on the existence of the variable on the right-hand side, so the JavaScript engine expects an object. Otherwise, we get the following error:
const { name, color } = undefined; // TypeError: Cannot destructure property 'name' of 'undefined' as it is undefined.
As a result, if you use named parameters with default values in the function declaration and the user does not provide at least one parameter assignment, the code throws an error.
function greet({ salutation = "Hello", name = "World" }) {
console.info(`${salutation} ${name}`);
}
greet(); // ERROR: Cannot read properties of undefined. See Example #3
greet({}); // Hello World
Assigning an empty object as a default parameter value is a better way to avoid this error.
function greet({ salutation = "Hello", name = "World" } = {}) {
console.info(`${salutation} ${name}`);
}
greet(); // Hello World
Using Previous Arguments in Later Default Parameters
We can use previous parameters while setting default values of the function parameters because parameter values are set left-to-right. Later default parameters may use the earlier parameters by simply using parameter names in their default value expression. See the following function for example:
function createPost(language = "en", title = translate(language, "Untitled") {
// Function body
}
const postA = createPost();
const postA = createPost("es");
The first parameter takes the language as an argument and uses English as a default value, and the second parameter, title
, sets its default value based on the first parameter.
Basic Philosophy
In software development, it is more important to know why than how. More sources explain the philosophy of the default values from an academic perspective, but I will explain the default values' importance in simple terms.
One of the most fundamental truths known to every web developer and UI designer is that people don't like filling out forms. This knowledge is so widespread that when I queried Google with the 'design better forms' search on the date I'm writing this, it returned a staggering 1,860,000,000 (almost 2 billion) results. Our users are other developers, including ourselves. So, do our users any less? An integral part of providing a high-quality developer experience, a suitable function interface is the cornerstone of a high-quality Application Programming Interface (API), especially if they are public API.
Sometimes, no matter what we do, we may need to use many parameters in functions, but even if we use a single parameter, default function parameters are necessary for a good developer experience.
Using default function parameters in function definition has several benefits:
- It's a cool trick to preserve backward compatibility while adding new parameters. (I suggest not to overuse this.)
- It decreases the mental burden of the users because they don't have to know what every and each parameter does. It provides a low entry barrier because each parameter is a brick on the entry barrier wall.
- It provides simplicity and makes complexity possible. Users start to use your function, and if later they need advanced features, they can use the related parameters accordingly.
- Default arguments increase the consistency of a program.
- It reduces the number of key presses of our users.
As you see, except for backward compatibility, using JavaScript default parameters boils down to developer experience.
Let's share your examples and thoughts in the comments below.
Comments ()